I’m very proud and excited to announce the release of PGPainless version 0.2! Since the last stable release of my OpenPGP library for Java and Android 9 months ago, a lot has changed and improved! Most importantly development on PGPainless is being financially sponsored by FlowCrypt, so I was able to focus a lot more energy into working on the library. I’m very grateful for this opportunity 🙂
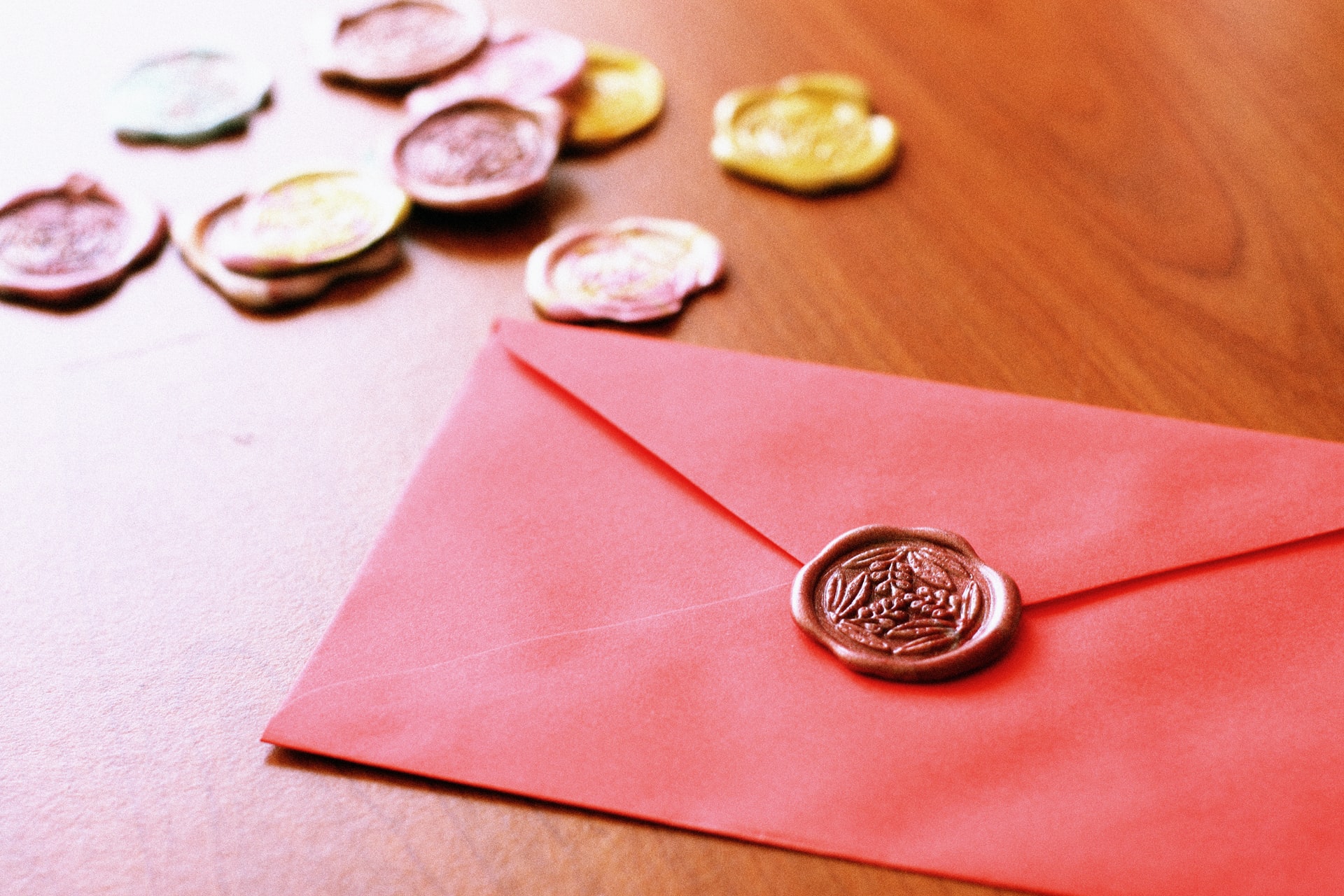
PGPainless is using Bouncycastle, but aims to save developers from the pain of writing lots of boilerplate code, while at the same time using the BC API properly. The new release is available on maven central, the source code can be found on Github and Codeberg.
PGPainless is now depending on Bouncycastle 1.68 and the minimum Android API level has been raised to 10 (Android 2.3.3). Let me bring you up to speed about some of its features and the recent changes!
Inspect Keys!
Back in the last stable release, PGPainless could already be used to generate keys. It offered some shortcut methods to quickly generate archetypal keys, such as simple RSA keys, or key rings based on elliptic curves. In version 0.2, support for additional key algorithms was added, such as EdDSA or XDH.
The new release introduces PGPainless.inspectKeyRing(keyRing)
which allows you to quickly access information about a key, such as its user-ids, which subkeys are encryption capable and which can be used to sign data, their expiration dates, algorithms etc.
Furthermore this feature can be used to evaluate a key at a certain point in time. That way you can quickly check, which key flags or algorithm preferences applied to the key 3 weeks ago, when that key was used to create that signature you care about. Or you can check, which user-ids your key had 5 years ago.
Edit Keys!
Do you already have a key, but want to extend its expiration date? Do you have a new Email address and need to add it as a user-id to your key? PGPainless.modifyKeyRing(keyRing)
allows basic modification of a key. You can add additional user-ids, adopt subkeys into your key, or expire/revoke existing subkeys.
secretKeys = PGPainless.modifyKeyRing(secretKeys) .setExpirationDate(expirationDate, keyRingProtector) .addSubKey(subkey, subkeyProtector, keyRingProtector) .addUserId(UserId.onlyEmail("alice@pgpainless.org"), keyRingProtector) .deleteUserId("alice@pgpainful.org", keyRingProtector) .revokeSubkey(subKeyId, keyRingProtector) .revokeUserId("alice@pgpaintrain.org", keyRingProtector) .changePassphraseFromOldPassphrase(oldPass) .withSecureDefaultSettings().toNewPassphrase(newPass) .done();
Encrypt and Sign Effortlessly!
PGPainless 0.2 comes with an improved, simplified encryption/signing API. While the old API was already quite intuitive, I was focusing too much on the code being “autocomplete-friendly”. My vision was that the user could encrypt a message without ever having to read a bit of documentation, simply by typing PGPainless and then following the autocomplete suggestions of the IDE. While the result was successful in that, the code was not very friendly to bind to real-world applications, as there was not one builder class, but several (one for each “step”). As a result, if a user wanted to configure the encryption dynamically, they would have to keep track of different builder objects and cope with casting madness.
// Old API EncryptionStream encryptionStream = PGPainless.createEncryptor() .onOutputStream(targetOuputStream) .toRecipient(aliceKey) .and() .toRecipient(bobsKey) .and() .toPassphrase(Passphrase.fromPassword("password123")) .usingAlgorithms(SymmetricKeyAlgorithm.AES_192, HashAlgorithm.SHA256, CompressionAlgorithm.UNCOMPRESSED) .signWith(secretKeyDecryptor, aliceSecKey) .asciiArmor(); Streams.pipeAll(plaintextInputStream, encryptionStream); encryptionStream.close();
The new API is still intuitive, but at the same time it is flexible enough to be modified with future features. Furthermore, since the builder has been divided it is now easier to integrate PGPainless dynamically.
// New shiny 0.2 API EncryptionStream encryptionStream = PGPainless.encryptAndOrSign() .onOutputStream(outputStream) .withOptions( ProducerOptions.signAndEncrypt( new EncryptionOptions() .addRecipient(aliceKey) .addRecipient(bobsKey) // optionally encrypt to a passphrase .addPassphrase(Passphrase.fromPassword("password123")) // optionally override symmetric encryption algorithm .overrideEncryptionAlgorithm(SymmetricKeyAlgorithm.AES_192), new SigningOptions() // Sign in-line (using one-pass-signature packet) .addInlineSignature(secretKeyDecryptor, aliceSecKey, signatureType) // Sign using a detached signature .addDetachedSignature(secretKeyDecryptor, aliceSecKey, signatureType) // optionally override hash algorithm .overrideHashAlgorithm(HashAlgorithm.SHA256) ).setAsciiArmor(true) // Ascii armor ); Streams.pipeAll(plaintextInputStream, encryptionStream); encryptionStream.close();
Verify Signatures Properly!
The biggest improvement to PGPainless 0.2 is improved, proper signature verification. Prior to this release, PGPainless was doing what probably every other Bouncycastle-based OpenPGP library was doing:
PGPSignature signature = [...]; // Initialize the signature with the public signing key signature.init(pgpContentVerifierBuilderProvider, signingKey); // Update the signature with the signed data int read; while ((read = signedDataInputStream.read()) != -1) { signature.update((byte) read); } // Verify signature correctness boolean signatureIsValid = signature.verify();
The point is that the code above only verifies signature correctness (that the signing key really made the signature and that the signed data is intact). It does however not check if the signature is valid.
Signature validation goes far beyond plain signature correctness and entails a whole suite of checks that need to be performed. Is the signing key expired? Was it revoked? If it is a subkey, is it bound to its primary key correctly? Has the primary key expired or revoked? Does the signature contain unknown critical subpackets? Is it using acceptable algorithms? Does the signing key carry the SIGN_DATA
flag? You can read more about why signature verification is hard in my previous blog post.
After implementing all those checks in PGPainless, the library now scores second place on Sequoia’s OpenPGP Interoperability Test Suite!
Lastly, support for verification of cleartext-signed messages such as emails was added.
New SOP module!
Also included in the new release is a shiny new module: pgpainless-sop
This module is an implementation of the Stateless OpenPGP Command Line Interface specification. It basically allows you to use PGPainless as a command line application to generate keys, encrypt/decrypt, sign and verify messages etc.
$ # Generate secret key $ java -jar pgpainless-sop-0.2.0.jar generate-key "Alice <alice@pgpainless.org>" > alice.sec $ # Extract public key $ java -jar pgpainless-sop-0.2.0.jar extract-cert < alice.sec > alice.pub $ # Sign some data $ java -jar pgpainless-sop-0.2.0.jar sign --armor alice.sec < message.txt > message.txt.asc $ # Verify signature $ java -jar pgpainless-sop-0.2.0.jar verify message.txt.asc alice.pub < message.txt $ # Encrypt some data $ java -jar pgpainless-sop-0.2.0.jar encrypt --sign-with alice.sec alice.pub < message.txt > message.txt.asc $ # Decrypt ciphertext $ java -jar pgpainless-sop-0.2.0.jar decrypt --verify-with alice.pub --verify-out=verif.txt alice.sec < message.txt.asc > message.txt
The primary reason for creating this module though was that it enables PGPainless to be plugged into the interoperability test suite mentioned above. This test suite uncovered a ton of bugs and shortcomings and helped me massively to understand and interpret the OpenPGP specification. I can only urge other developers who work on OpenPGP libraries to implement the SOP specification!
Upstreamed changes
Even if you are not yet convinced to switch to PGPainless and want to keep using vanilla Bouncycastle, you might still benefit from some bugfixes that were upstreamed to Bouncycastle.
Every now and then for example, BC would fail to do some internal conversions of elliptic curve encryption keys. The source of this issue was that BC was converting keys from BigInteger
s to byte array
s, which could be of invalid length when the encoding was having leading zeros, thus omitting one byte. Fixing this was easy, but finding the bug was taking quite some time.
Another bug caused decryption of messages which were encrypted for more than one key/passphrase to fail, when BC tried to decrypt a Symmetrically Encrypted Session Key Packet with the wrong key/passphrase first. The cause of this issue was that BC was not properly rewinding the decryption stream after reading a checksum, thus corrupting decryption for subsequent attempts with the correct passphrase or key. The fix was to mark and rewind the stream properly before the next decryption attempt.
Lastly some methods in BC have been modernized by adding generics to Iterator
s. Chances are if you are using BC 1.68, you might recognize some changes once you bump the dependency to BC 1.69 (once it is released of course).
Thank you!
I would like to thank anyone who contributed to the new release in any way or form for their support. Special thanks goes to my sponsor FlowCrypt for giving me the opportunity to spend so much time on the library! Furthermore I’d like to thank the all the amazing folks over at Sequoia-PGP for their efforts of improving the OpenPGP ecosystem and patiently helping me understand the (at times at bit muddy) OpenPGP specification.
3 responses to “PGPainless 0.2 Released!”
@vanitasvitae congrats on the release!
@vanitasvitae That's a great blog describing why signature verification is nontrivial. Thanks for posting! #pgp
[…] "I’m very proud and excited to announce the release of #PGPainless version 0.2! Since the last stable release of my #OpenPGP library for Java and Android 9 months ago, a lot has changed and improved! Most importantly development on PGPainless is being financially sponsored" https://blog.jabberhead.tk/2021/06/02/pgpainless-0-2-released/ […]